You landed here because JV-PF is a replacement for the following:
JV-100: Java for New Programmers
GOT IT!
Questions? Call 602-266-8585
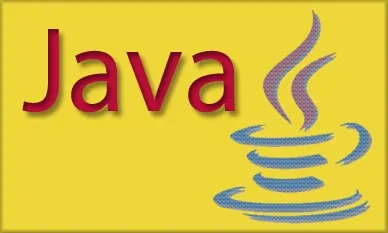
x
Course: Outline
Module 1 Essential skills
- An introduction to Java
- How to write your first Java applications
- How to work with the primitive data types
- How to code control statements
- How to code methods, handle exceptions, and validate data
- How to test, debug, and deploy an application
Module 2 Object-oriented programming
- How to define and use classes
- How to work with inheritance
- How to define and use interfaces
- More object-oriented programming skills
Module 3 More essential skills
- How to work with arrays
- How to work with collections and generics
- How to work with strings
- How to work with dates and times
- How to work with file I/O
- How to work with exceptions
Module 4 GUI programming
- How to get started with JavaFX
- How to get started with Swing
- More Swing controls
Module 5 Database programming
- An introduction to databases with SQLite
- How to use JDBC to work with a database
Module 6 Advanced skills
- How to work with lambda expressions and streams
- How to work with threads
Audience
Programmers who need to design and develop Java applets and applications.
Prerequisites
Students taking this course must be able to operate a PC and have a basic understanding of such programming concepts as simple variables, sequence of code statements, looping, decisions (e.g. if statement), directory navigation, and files. Object oriented programming experience is not required for this course nor is web development.
What You Will Learn
- Create and explain project structure in Eclipse
- Work with classes, methods, statements, and class attributes
- Correct syntax and logic errors using the debugging tools in Eclipse
- Work with Java primitive datatypes and Java data classes
- Apply various levels of visibility to classes, subclasses, packages, and instances
- Code three types of loops, various conditional statements, use case statements
- Work with Java exceptions
- Use interfaces and polymorphism to create decoupled objects
- Apply basic design patterns to create well structured code
- Read and write to a file
- Use simple JDBC to manipulate a database